Using tools
Tools on a tool bar can be used much like widgets. Resources such as width, height, borderWidth, foregroundColor, and backgroundColor can control the appearance of the tool. The variableWidth resource controls whether a tool shrinks or grows as the tool bar resizes.
The enterNotifyCallback and leaveNotifyCallback can determine when the mouse enters or leaves the location on the tool bar occupied by a tool. These callbacks are useful for implementing features such as bubble help or tool tips or for updating a status area.
Using primitive tools
The most commonly used tools on a tool bar are
primitive tools. These are tools that behave much like simple widgets such as buttons and labels. Primitive tools have an image which determines the text or graphics that they display. The image can be any renderable object (see
Renderables).
Label tools (EwLabelTool) display an image and provide a shadowed inset as specified in the shadowType resource. They provide resources for setting margins similar to the CwLabel widget. Progress bar tools (EwProgressBarTool) look and behave much like EwProgressBar. The button tools (EwPushButtonTool and EwToggleButtonTool) also behave much like their CwWidget counterparts. A separator tool (EwSeparatorTool) can separate tools or clusters of tools. The separatorType resource determines the exact appearance of a separator tool.
The following method creates a tool bar with four primitive tools. Add this method to a class using the pool dictionaries CwConstants and EwConstants.
toolBarExample
| shell toolBar |
shell := CwTopLevelShell
createApplicationShell: 'Tool Bar Example'
argBlock: nil.
toolBar := EwToolBar
createManagedWidget: 'toolBar'
parent: shell
argBlock: [:w | w numColumns: 0; spacing: 3].
toolBar
createLabelTool: 'label'
argBlock: [:w | w image: 'Text Label'].
toolBar
createSeparatorTool: 'label'
argBlock: [:w | w
orientation: XmVERTICAL;
autoSize: true;
separatorType: XmSHADOWETCHEDIN].
toolBar
createProgressBarTool: 'progressBar'
argBlock: [:w | w fractionComplete: 1/2].
toolBar
createPushButtonTool: 'pushButton'
argBlock: [:w | w image: 'Push Me'; width: 80].
shell realizeWidget.
When you run the method by evaluating <class name> new toolBarExample, you get the following:
Using widget tools
Widgets can be added to a tool bar by creating a widget tool. A widget tool can be created by sending the createWidgetTool:name:argBlock: message to the toolbar. When adding a widget tool, the application specifies the class of widget to be created.
The argument for a widget tool argBlock is the tool itself, not the widget. To set resources for the tool's associated widget, use the widget resource to access the widget from the tool.
The following example creates a widget tool using a combo box. It uses the argument block to set both widget and tool resources.
...
toolBar createWidgetTool: CwComboBox name: 'combo' argBlock: :tool |
tool
borderWidth: 1;
height: 36.
tool widget
items: #('Red' 'Green' 'Blue');
editable: true].
...
Using groups
Groups (EwGroupTool) are useful for grouping multiple tools in order to assign common properties or provide specialized behavior for the group. The shadowType resource determines what kind of border is drawn around the tools within a group when the borderWidth is not 0. The spacing resource determines what spacing the group uses for its tools. If the group contains toggle button tools, the radioBehavior resource can enforce radio button style behavior within a group.
A group is created like any other tool--by sending either createGroup:argBlock: or createSimpleGroup:argBlock: to the toolbar. Tools are added to the group by sending tool creation messages to the group instead of the tool bar.
The following method below creates a tool bar that contains two groups separated by a separator tool.
toolGroupExample
| shell toolBar group1 group2 |
shell := CwTopLevelShell
createApplicationShell: 'Tool Group Example'
argBlock: nil.
toolBar := EwToolBar
createManagedWidget: 'toolBar'
parent: shell
argBlock: :w | w numColumns: 0; spacing: 3].
group1 := toolBar
createGroup: 'group1'
argBlock: :w | w borderWidth: 1; shadowType: XmSHADOWIN].
group1
createLabelTool: 'label'
argBlock: :w | w image: 'Text Label'].
group1
createProgressBarTool: 'progressBar'
argBlock: :w | w fractionComplete: 1/2].
toolBar
createSeparatorTool: 'label'
argBlock: :w | w
orientation: XmVERTICAL;
autoSize: true;
separatorType: XmSHADOWETCHEDIN].
group2 := toolBar
createGroup: 'group2'
argBlock: :w | w
borderWidth: 1;
shadowType: XmSHADOWOUT;
radioBehavior: true].
group2
createToggleButtonTool: 'toggle1'
argBlock: :w | w image: 'Yes'; radioBehavior: true].
group2
createToggleButtonTool: 'toggle2'
argBlock: :w | w image: 'No'; radioBehavior: true; set: true].
shell realizeWidget.
Evaluating
<class name> new toolGroupExample displays the following:
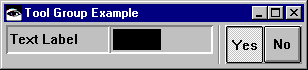
Simple groups (EwSimpleGroupTool) provide convenience methods for creating groups of buttons by simply specifying a collection of images from which button tools will be created. The buttonBorderWidth, radioBehavior, and toggleBehavior resources specify the appearance and behavior of the buttons.
The example below creates a group like the radio group in the previous example, but it uses a simple group to create the toggle buttons.
...
group2 := toolBar createSimpleGroup: 'group2' argBlock: :w | w
borderWidth: 1;
shadowType: XmSHADOWOUT;
radioBehavior: true;
toggleBehavior: true;
images: #('Yes' 'No')].
...