SelectionBox widgets
Selection-box widgets (CwSelectionBox) are used to obtain a selection from a list of alternatives provided to the user. A selection box can contain some or all of the following components: a scrollable list of alternatives, an editable text field for the selected alternative, labels for the list and text field, and four buttons. By default, the buttons are labelled OK, Apply, Cancel and Help.
A selection box is usually created in a dialog shell to prompt the user to select a list item. Selection-box widgets are created using the createSelectionBox:argBlock: and createSelectionDialog:argBlock: convenience methods. The latter method is similar to the first, but also creates a CwDialogShell as the parent of the CwSelectionBox so that it can be popped up separately from the main widget tree. As with pop-up menus a selection dialog can be created and left unmanaged until it is to be presented to the user. It is popped up by sending the selection box the manageChild message. The default behavior is to remain open until either the OK or Cancel button is pressed, or the dialog's close box is double-clicked. The application can explicitly close the dialog by sending unmanageChild to the selection box.
The dialogType resource specifies which selection-box components actually appear. It can have one of the following values:
XmDIALOGPROMPT
All standard children except the list and list label are created and all except the Apply button are managed.
XmDIALOGSELECTION
All standard children are created and managed. This is the default if the parent is a dialog shell.
XmDIALOGWORKAREA
All standard children are created, and all except the Apply button are managed. This is the default if the parent is not a dialog shell.
When the selection box is in a dialog shell, the dialogTitle resource specifies the title of the dialog shell, and the dialogStyle resource specifies the input mode while the dialog is active.
The labels for the list widget and the text widget are set using the selectionLabelString: and textLabelString: methods respectively.
An example selection dialog is shown in the diagram below.
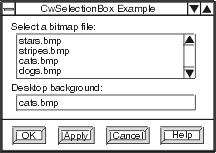
The list contents can be set or retrieved using the listItems resource. The text contents can be set or retrieved using the textString resource.
The widgets comprising the selection box can be retrieved using the getChild: method. If a button or other widget is not required by the application, it can be retrieved and unmanaged. Possible arguments to the getChild: method are:
•XmDIALOGAPPLYBUTTON
•XmDIALOGCANCELBUTTON
•XmDIALOGDEFAULTBUTTON
•XmDIALOGHELPBUTTON
•XmDIALOGLIST
•XmDIALOGLISTLABEL
•XmDIALOGOKBUTTON
•XmDIALOGSELECTIONLABEL
•XmDIALOGSEPARATOR
•XmDIALOGTEXT
•XmDIALOGWORKAREA
The okCallback, applyCallback, cancelCallback, and helpCallback of the selection box are run when the corresponding buttons are pressed. If a callback is not added, the corresponding button will still appear, but nothing will happen when it is pressed. When the selection box is in a dialog shell, it is unmanaged when the OK or Cancel button is pressed, unless the autoUnmanage resource is set to false.
Using the mustMatch resource, the selection box can be configured to test whether the text typed in the text widget matches any item in the list. If mustMatch is true and the text does not match any item in the list when the OK button is pressed, the noMatch callback is run, otherwise the ok callback is run. Note that if the noMatch callback is run, a selection dialog will not be unmanaged.
The code below creates the following
modeless selection dialog that is popped up when a button is pressed. The name of the Apply button has been changed to Show, and the Help button is hidden.
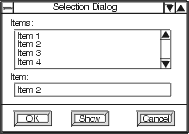
The mustMatch resource and noMatch callback are used to test when the text does not match any item in the list. Because the dialog is modeless, the user can click or type in any other widget without having to close the dialog first.
Object subclass: #SelectionDialogExample
instanceVariableNames: 'selectionBox '
classVariableNames: ''
poolDictionaries: 'CwConstants'
open
| items shell button |
items := OrderedCollection new.
1 to: 20 do: [:i |
items add: 'Item ', i printString].
shell := CwTopLevelShell
createApplicationShell: 'shell'
argBlock: [:w | w title: 'Selection Dialog Example'].
button := shell
createPushButton: 'Push me for selection box'
argBlock: nil.
button
addCallback: XmNactivateCallback
receiver: self
selector: #button:clientData:callData:
clientData: nil.
button manageChild.
selectionBox := shell
createSelectionDialog: 'selection'
argBlock: [:w |
w
dialogTitle: 'Selection Dialog';
dialogStyle: XmDIALOGMODELESS;
dialogType: XmDIALOGSELECTION;
listLabelString: 'Items:';
selectionLabelString: 'Selection:';
applyLabelString: 'Show';
listItems: items;
mustMatch: true].
selectionBox
addCallback: XmNokCallback
receiver: self
selector: #ok:clientData:callData:
clientData: nil;
addCallback: XmNapplyCallback
receiver: self
selector: #show:clientData:callData:
clientData: nil;
addCallback: XmNcancelCallback
receiver: self
selector: #cancel:clientData:callData:
clientData: nil;
addCallback: XmNnoMatchCallback
receiver: self
selector: #noMatch:clientData:callData:
clientData: nil.
(selectionBox getChild: XmDIALOGHELPBUTTON) unmanageChild.
shell realizeWidget
button: widget clientData: clientData callData: callData
"The button has been pressed. Pop up the selection dialog."
selectionBox manageChild
ok: widget clientData: clientData callData: callData
"The OK button in the dialog has been pressed.
The dialog will be automatically unmanaged."
Transcript cr; show: 'The final selection is: ' , widget textString
show: widget clientData: clientData callData: callData
"The Show button in the dialog has been pressed.
The dialog will NOT be automatically unmanaged."
Transcript cr; show: widget textString
cancel: widget clientData: clientData callData: callData
"The Cancel button in the dialog has been pressed.
The dialog will be automatically unmanaged."
Transcript cr; show: 'The selection dialog was canceled.'
noMatch: widget clientData: clientData callData: callData
"The OK button in the dialog was pressed,
but the textString did not match any item in
the items list. The dialog will NOT be automatically unmanaged."
Transcript cr; show: 'The selection does not match any item in the list.'