MessageBox widgets
Message-box widgets (CwMessageBox) are used for common interaction tasks such as displaying messages, asking questions and reporting errors. A message box widget consists of a message string, an optional symbol such as an exclamation or question mark, and three buttons. By default, the buttons are labelled OK, Cancel, and Help, and there is no symbol displayed.
A message box is usually created in a dialog shell to notify the user of some event. Message-box widgets are created using the createMessageBox:argBlock: and createMessageDialog:argBlock: convenience methods. The latter method is similar to the first, but also creates a CwDialogShell as the parent of the CwMessageBox so that it can be popped up separately from the main widget tree. As with pop-up menus, the message-box/dialog shell combination (message dialog) can be created and left unmanaged until it is to be presented to the user. It is popped up by sending the message box the manageChild message. The default behavior is to remain open until either the OK or Cancel button is pressed, or the dialog's close box is double-clicked. The application can explicitly close the dialog by sending unmanageChild to the message box.
The message shown in a message box is specified by the messageString resource. When the message box is in a dialog shell, the dialogTitle resource specifies the title of the dialog shell. The symbol shown is specified by the dialogType resource, which can have one of the following values:
XmDIALOGMESSAGE
Message only, no symbol (default)
XmDIALOGINFORMATION
Information symbol (an i on some plaforms)
XmDIALOGERROR
Error symbol (a stop sign on some platforms)
XmDIALOGWARNING
Warning symbol (an ! on some platforms)
XmDIALOGQUESTION
Question mark symbol
XmDIALOGWORKING
Working symbol
The following figure shows an example message dialog:
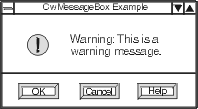
The dialogStyle resource specifies the input mode while the dialog is active, and can have one of the following values:
XmDIALOGAPPLICATIONMODAL
Used for dialogs that must be responded to before some other interactions in ancestors of the widget. This value is the same as XmDIALOG_PRIMARY_APPLICATION_MODAL and remains for compatibility.
XmDIALOGWORKAREA
Used for BulletinBoard widgets whose parents are not DialogShells. This is the default when the parent of the BulletinBoard is not a DialogShell.
XmDIALOGMODELESS
Used for dialogs that do not interrupt interaction of any application (default).
XmDIALOGPRIMARYAPPLICATIONMODAL
Used for dialogs that must be responded to before any other interaction in ancestors of the widget.
XmDIALOGFULLAPPLICATIONMODAL
Used for dialogs that must be responded to before any other interaction in the same application.
XmDIALOGSYSTEMMODAL
Used for dialogs that must be responded to before any other interaction in any application.
If a platform does not support the specified prompter style, the style is promoted to the next most restrictive style. If the next most restrictive style is not supported, the style is demoted to the next less restrictive style.
The widgets comprising the message box can be retrieved using the getChild: method. If a button or other widget is not required by the application, it can be retrieved and unmanaged. Possible arguments for the getChild: method are:
•XmDIALOGCANCELBUTTON
•XmDIALOGDEFAULTBUTTON
•XmDIALOGHELPBUTTON
•XmDIALOGMESSAGELABEL
•XmDIALOGOKBUTTON
•XmDIALOGSEPARATOR
•XmDIALOGSYMBOLLABEL
The okCallback, cancelCallback, and helpCallback of the message box are called when the corresponding buttons are pressed. If a callback is not added, the corresponding button will still appear, but nothing will happen when it is pressed. If the message box is in a dialog shell, it is unmanaged when the OK or Cancel button is pressed, unless the autoUnmanage resource is set to false.
The following code creates an application modal information message dialog that is popped up when a button is pressed. Only the OK button of the message dialog is shown. The remaining ones are hidden (unmanaged). A callback is added for the OK button. Because the dialog is application modal, the user must close the dialog before the application can receive further input.
Object subclass: #MessageDialogExample
instanceVariableNames: 'messageBox '
classVariableNames: ''
poolDictionaries: 'CwConstants'
open
| shell button |
shell := CwTopLevelShell
createApplicationShell: 'shell'
argBlock: [:w | w title: 'Message Dialog Example'].
button := shell
createPushButton: 'Push me for dialog'
argBlock: nil.
button
addCallback: XmNactivateCallback
receiver: self
selector: #button:clientData:callData:
clientData: nil.
button manageChild.
messageBox := shell
createMessageDialog: 'message'
argBlock: [:w |
w
dialogTitle: 'Message Dialog';
dialogStyle: XmDIALOGFULLAPPLICATIONMODAL;
messageString: 'This is a message dialog.';
dialogType: XmDIALOGINFORMATION].
messageBox
addCallback: XmNokCallback
receiver: self
selector: #ok:clientData:callData:
clientData: nil.
(messageBox getChild: XmDIALOGCANCELBUTTON) unmanageChild.
(messageBox getChild: XmDIALOGHELPBUTTON) unmanageChild.
shell realizeWidget
The activate and OK callbacks used by the code is shown below.
button: widget clientData: clientData callData: callData
"The button has been pressed. Pop up the message dialog."
messageBox manageChild
ok: widget clientData: clientData callData: callData
Transcript
cr; show: 'The OK button in the dialog has been pressed.';
cr; show: 'The dialog is about to disappear.'.